Omniauth Authentication Ruby on Rails
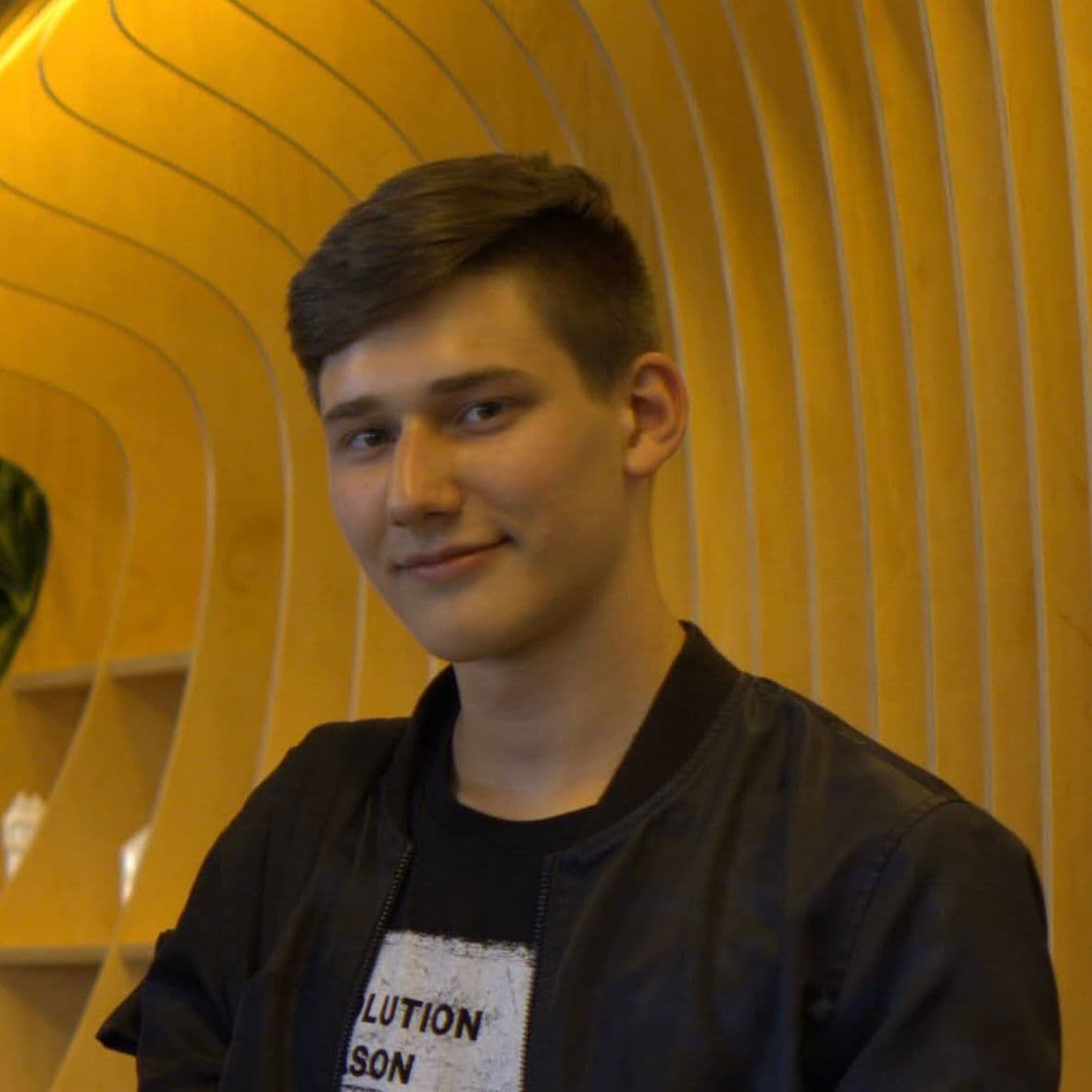
Aleksandr Riabov
Jul 7, 2023
How to make authorization on Ruby on Rails with Omniauth?
To implement a simple authorization using Omniauth in Ruby on Rails, follow these steps:
- Add dependencies to Gemfile
- Create GitHub app
- Create new file omniauth.rb
- Add button and route
- Create sessions
Add dependencies to Gemfile:
Open your Gemfile and add the following lines:
gem 'omniauth-github', github: 'omniauth/omniauth-github', branch: 'master'
gem "omniauth-rails_csrf_protection"
Save the Gemfile and run '
Create GitHub app:
To use GitHub as the authentication provider, you need to create a GitHub app. Follow these steps:
- Go to your GitHub account settings.
- Navigate to "Developer Settings" and click on "OAuth Apps".
- Click on "New OAuth App" to create a new app.
- Fill in the required details, including your website's URL and the authorization callback URL. Use your website's URL with "/auth/github/callback" appended to it, instead of "localhost:3000" if you are deploying on production.
Save the app settings.
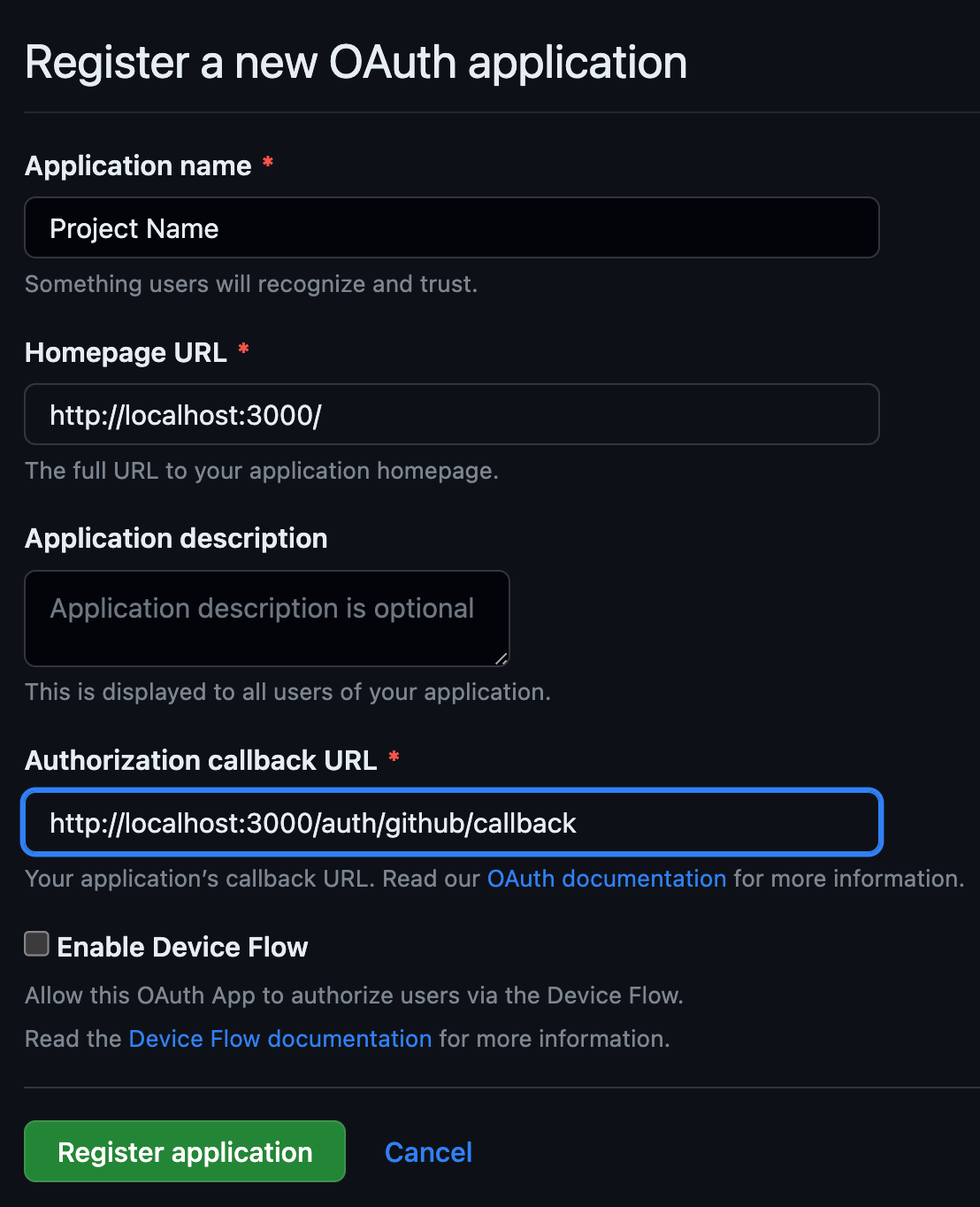
Create new file omniauth.rb:
In the 'config/initializers' folder, create a new file called 'omniauth.rb' and add the following code:
Rails.application.config.middleware.use OmniAuth::Builder do
provider :github, ENV['GITHUB_CLIENT_ID'], ENV['GITHUB_CLIENT_SECRET']
end
This code initializes the OmniAuth middleware and configures the GitHub provider with your client ID and client secret. Make sure to set the 'GITHUB_CLIENT_ID' and 'GITHUB_CLIENT_SECRET' environment variables in your application.
Add a button and route:
In your 'app/views/layouts/application.html.erb file', add the following line of code where you want the "Sign in with GitHub" button to appear:
<%= button_to 'Sign in with GitHub', '/auth/github', data: { turbo: false } %>
This code creates a button that will trigger the authentication process with GitHub when clicked.
Next, open the 'config/routes.rb' file and add the following line:
get 'auth/github/callback', to: 'sessions#create'
This route maps the callback URL to the 'create' action in the 'SessionsController'.
Create sessions:
Create a new file called 'sessions_controller.rb' in the 'app/controllers' folder and add the following code:
class SessionsController < ApplicationController
def create
auth_hash = request.env['omniauth.auth']
print(auth_hash)
redirect_to root_path
end
end
This code defines the create action in the SessionsController. Inside the action, the auth_hash variable stores the authentication information returned by GitHub. You can process this information as needed. In this example, we print the auth_hash and then redirect the user to the root path.